- Published on
- | 5 mins read
Chap 2 - Show a SVG Image in a Flame Game
- Authors
- Name
- Miller Go Dev
- @millergodev
Overview
Table of Contents
Install the Flame package
We navigate to the project root directory and run this command:
flutter pub add flame
It will add this line into the pubspec.yaml
file and download the package into our project.
dependencies:
...
freezed_annotation: ^2.1.0
json_annotation: ^4.7.0
+ flame: ^1.3.0
Then, we can create a new file named main_game.dart
in the lib
directory. This may not be the most proper place, but we can live with it for now.
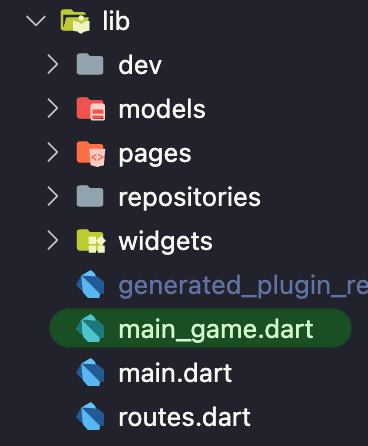
In this file, we declare our FlameGame
class. An instance of this class is the entry point to interact with our Flame game.
import 'package:flame/game.dart';
class MainGame extends FlameGame {}
It imported the FlameGame
class from the game.dart
library. That means we added the flame
package successfully.
Show the game
Our MainGame
is just a normal Dart class. It needs to be instantiated somewhere and attached to a Flutter widget to be able to be seen on the screen. Luckily, flame
provides a GameWidget
to hold the FlameGame
instance.
GameWidget(game: MainGame())
Now, where do we want to interact with our game, with the Monopoly cards? It should be after players joined the game room. In the current flow, that's the place of the GamePage
widget. So all we need to do is to find to the GamePage
instance in main.dart
file and replace it with our GameWidget
.
+import 'package:flame/game.dart';
import 'package:flutter/material.dart';
import 'package:monopoly_deal/dev/repositories.dart';
+import 'package:monopoly_deal/main_game.dart';
import 'package:monopoly_deal/pages/game_page.dart';
import 'package:monopoly_deal/repositories/game_repository.dart';
import 'package:monopoly_deal/routes.dart';
...
@override
Widget build(BuildContext context) {
return MaterialApp(
initialRoute: AppRoutes.home,
routes: {
AppRoutes.home: (_) => HomePage(gameRepository: gameRepository),
- AppRoutes.game: (_) => GamePage(gameRepository: gameRepository),
+ AppRoutes.game: (_) => GameWidget(game: MainGame()),
},
);
}
Let's run the game!
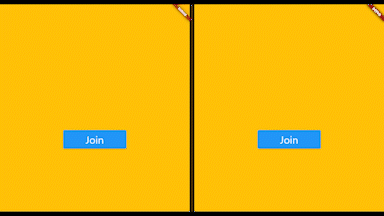
Show the pause button
It's an empty game, that's why we only see a black screen. Now, we want to show a pause button so that user can tap on it later to view the pause menu and go back to the home page.
Let's grab a free pause icon from IconPark. A good source for getting common beautiful icons. We download it as a SVG and save in the assets/images
directory.
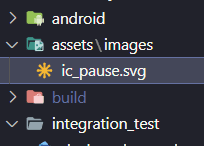
And remember to register that path in the pubspec.yaml
file. Otherwise, it won't work.
# To add assets to your application, add an assets section, like this:
- # assets:
- # - images/a_dot_burr.jpeg
- # - images/a_dot_ham.jpeg
+ assets:
+ - assets/images/
We also need to add the flame_svg
package. Otherwise, we can't load a SVG image. Run this command in the project's root directory:
flutter pub add flame_svg
And your pubspec.yaml
file should have this line:
dependencies:
equatable: ^2.0.5
freezed_annotation: ^2.1.0
json_annotation: ^4.7.0
flame: ^1.3.0
+ flame_svg: ^1.5.0
Now, we need to load this image in our game. We want to do it right after our game started. Luckily, Flame engine has this onLoad()
callback that is called once the game begins. So, we need to override it in our MainGame
class.
class MainGame extends FlameGame {
Color backgroundColor() => const Color(0xFFFFFFFF);
Future<void>? onLoad() async {
final pauseIcon = await Svg.load('images/ic_pause.svg');
final pauseIconComponent = SvgComponent(
svg: pauseIcon,
position: Vector2.all(0),
size: Vector2.all(100),
);
await add(pauseIconComponent);
}
}
In this function, we called the static load()
function to get the Svg
instance referring to our icon. Then, we instantiated a Component
of Flame engine.
A component is an object that holds some information about an UI component in our game. The FlameGame
will use that information to know how to draw it on the screen. You can also learn more about components of Flame here.
In this case, an SvgComponent
that is presented by a SVG image will be displayed at position
(0,0), which means the top left corner, and it has the size
of 100 in both sides.
After the component instance is created, we must call the add()
function to actually insert it into the game screen.
We also overridden the backgroundColor()
function to set background color for our game to be white. Otherwise, we cannot see the pause icon because it is also black.
Now, let's run the game.
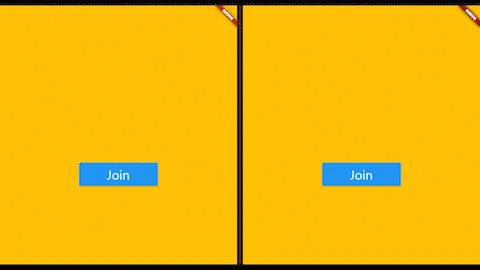
It's good.
Check out the commit for this article in this pull request.